|
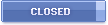 |
08-08-2005, 11:27 AM
|
#1
|
Knows Where the Search Button Is
Join Date: Jul 2005
Location: Washington
Model: 8300
Carrier: AT&T
Posts: 29
|
Data Save & Read Example
Please Login to Remove!
Just for the folks that are getting started with BB development, here is some sample code of a small app that demonstrates how to save and read data using the BB api for Persistent storage, along with using screen fields and buttons. I'm sure that some of the other developers here can offer some further tips on making this snipet better - but at least it's a good start for new BB developers. Enjoy:
Code:
/*
* DataSave.java
* © Timothy Trimble, 2005
* www.timothytrimble.info
* Demonstrates how to save and retrieve data
*/
import net.rim.device.api.ui.*;
import net.rim.device.api.ui.component.*;
import net.rim.device.api.ui.container.*;
import net.rim.device.api.system.*;
import net.rim.device.api.i18n.*;
import net.rim.device.api.util.*;
import java.util.*;
// DataSave.java Class --------------------------------------------------------------------------
public class DataSave extends net.rim.device.api.ui.UiApplication implements FieldChangeListener
{
private AutoTextEditField inputField; //define the edit fields
private AutoTextEditField anotherField;
private LabelField appTitle; //application title label
private ButtonField saveButton; //define the buttons
private ButtonField getButton;
//string used for PersistentObject is: com.rim.samples.docs.datasave
//per the RIM docs, you have to create a unique id for the persistent object
//this is done by entering a string in IDE, select the string, right click on the string,
//then select 'Convert to long
//declare the PersistentObject handle
private static PersistentObject mystore;
static
{
mystore = PersistentStore.getPersistentObject( 0xe63e08aacd575338L );
}
public static void main(String[] args)
{
DataSave TIinstance = new DataSave(); //create an instance of this class app
TIinstance.enterEventDispatcher(); //define a handle for the event processor
}
public DataSave()
{
MainScreen inputScreen = new MainScreen(); //create screen instance
appTitle = new LabelField("Input Test2"); //define app title
inputField = new AutoTextEditField("Input1: ",""); //define edit fields
anotherField = new AutoTextEditField("Input2: ","");
saveButton = new ButtonField("Save Data"); //define button titles
getButton = new ButtonField("Get Data");
saveButton.setChangeListener(this); //define the button handles for the event processor
getButton.setChangeListener(this);
inputScreen.setTitle(appTitle); //post the screen title
inputScreen.add(inputField); //post the input fields on screen
inputScreen.add(anotherField);
inputScreen.add(saveButton); //post the buttons on the screen
inputScreen.add(getButton);
pushScreen(inputScreen); //post the screen itself
}
public void fieldChanged(Field field, int context) //respond to button events
{
if (field == saveButton) //if save data button selected
{
String myField1 = inputField.getText(); //read text from first field
String myField2 = anotherField.getText(); //read text from second field
String[] fieldInfo = {myField1, myField2}; //store text into string record
synchronized(mystore) //lock down this thread
{
mystore.setContents(fieldInfo); //store the string record
mystore.commit(); //commit the changes
}
Dialog.alert(inputField.getText() + " saved."); //show text from field
}
if (field == getButton) //if get data button selected
{
synchronized(mystore) //lock down this thread
{
String[] currentInfo = (String[])mystore.getContents(); //read the data
if (currentInfo == null) //if no data found
{
Dialog.alert("Data not found.");
}
else //if data is found
{
inputField.setText(currentInfo[0]); //get 1st string, put in screen field
anotherField.setText(currentInfo[1]); //get 2nd string, put in screen field
}
}
}
}
}
__________________
===================================
"There are 10 types of people in the world.
Those that understand binary and those that don't!"
www.timothytrimble.info - The ART of S/W Development
==================================
|
Offline
|
|
08-09-2005, 08:08 AM
|
#2
|
BlackBerry Extraordinaire
Join Date: Dec 2004
Location: in a house...
Model: lots
Carrier: Rogers
Posts: 1,148
|
No offense Tim, but maybe you should give credit to RIM for this example being that most of it is their code.
For everyone else, this is pretty much straight out of the samples with exception to the screen layout.
cd.
|
Offline
|
|
08-09-2005, 08:12 AM
|
#3
|
No longer Registered.
Join Date: Mar 2005
Location: Atlanta
Model: 8330
OS: 4.5.0.138
PIN: 31a6c9c9
Carrier: Verizon BIS
Posts: 13,962
|
lol corey I dont see him taking credit just posting it for others
|
Offline
|
|
08-09-2005, 10:17 AM
|
#4
|
Knows Where the Search Button Is
Join Date: Jul 2005
Location: Washington
Model: 8300
Carrier: AT&T
Posts: 29
|
Quote:
Originally Posted by corey@12mile
No offense Tim, but maybe you should give credit to RIM for this example being that most of it is their code.
For everyone else, this is pretty much straight out of the samples with exception to the screen layout.
cd.
|
Yes, a lot of the code is from the sample in the docs. I've mostly adapted it to the use of the buttons and screen fields. It's mostly for folks who come here looking for an example. Also, I've added a lot of comments to help explain what's going on.
__________________
===================================
"There are 10 types of people in the world.
Those that understand binary and those that don't!"
www.timothytrimble.info - The ART of S/W Development
==================================
|
Offline
|
|
08-09-2005, 10:59 AM
|
#5
|
BlackBerry Extraordinaire
Join Date: Dec 2004
Location: in a house...
Model: lots
Carrier: Rogers
Posts: 1,148
|
/*
* DataSave.java
* © Timothy Trimble, 2005
* www.timothytrimble.info
* Demonstrates how to save and retrieve data
*/
no?
|
Offline
|
|
08-09-2005, 01:33 PM
|
#6
|
Knows Where the Search Button Is
Join Date: Feb 2005
Location: New Mexico
Posts: 26
|
Hey Tim!
Keep on with the samples. I need them.
As for the originality critics, why not share an original idea of your own? It may just be harder to do than you think.
Again --- thanks!
|
Offline
|
|
08-09-2005, 01:43 PM
|
#7
|
BlackBerry Extraordinaire
Join Date: Dec 2004
Location: in a house...
Model: lots
Carrier: Rogers
Posts: 1,148
|
Hey Learner... how about this... piss off. you have no idea about originality.
|
Offline
|
|
08-09-2005, 02:16 PM
|
#8
|
Knows Where the Search Button Is
Join Date: Jul 2005
Location: Washington
Model: 8300
Carrier: AT&T
Posts: 29
|
Gents,
There's really no need to engage in a debate over this. To put more light on this, I did have the App Developer Guide (vol 2) open while I wrote this code snipit. However, it is not "straight" out of the docs. If there is such concern over the use of statements out of the published samples, then I'm sure that RIM would have taken issue with it a long time ago. The intent here is to share our knowledge, and thus aid those who are just getting started with BB development. BTW: My placement of (c) occurs with everthing I write. My only mistake here was including it in the pasted sample on this forum. By my placing it on this public forum, it is implied that anyone can copy and use it as needed. We're not talking rocket science or top secret calculations here. So, get over it and lets just be helpful instead of so critical.
__________________
===================================
"There are 10 types of people in the world.
Those that understand binary and those that don't!"
www.timothytrimble.info - The ART of S/W Development
==================================
|
Offline
|
|
08-09-2005, 03:29 PM
|
#9
|
New Member
Join Date: Aug 2005
Model: 7290
Posts: 5
|
Thank you for posting. Please don't let the negativity dissuade you from posting again!
I'm happy to finally see some activity on the developers forum, it has been quiet for a while.
|
Offline
|
|
08-09-2005, 03:43 PM
|
#10
|
Knows Where the Search Button Is
Join Date: Jun 2005
Model: 7520
Posts: 40
|
Can't we all just get along? lol!
This is good for the basic start up guys like me. I post code for my own reference and learning. Posting code can show flaws in your approach and may also garner kudos or crapos from other forum members. Usually the group can look and see what can be done optmize or improve the code. That's cool.
As far as credit, it's hard to put the basic stuff together without having it look like a rehash from the manual. I like how the timinatorolla commented the code and added some insight as to what the hell was going on  That was helpful.
I know I have a stash of code I have in a word doc that I plan on posting one day so all can learn, pick, crit, and comment.
Peace out.
|
Offline
|
|
08-09-2005, 03:45 PM
|
#11
|
Knows Where the Search Button Is
Join Date: Jun 2005
Model: 7520
Posts: 40
|
Timinator your picture cracks me up.
I think it's the hat.
|
Offline
|
|
08-09-2005, 04:56 PM
|
#12
|
Knows Where the Search Button Is
Join Date: Jul 2005
Location: Washington
Model: 8300
Carrier: AT&T
Posts: 29
|
Quote:
Originally Posted by BlackBerry Fairy
Timinator your picture cracks me up.
I think it's the hat.
|
Thanks.  I get a lot of comments on that. It's a left over from an old photo shoot I did when I was playing in a band.
__________________
===================================
"There are 10 types of people in the world.
Those that understand binary and those that don't!"
www.timothytrimble.info - The ART of S/W Development
==================================
|
Offline
|
|
08-09-2005, 09:41 PM
|
#13
|
Knows Where the Search Button Is
Join Date: Feb 2005
Location: New Mexico
Posts: 26
|
Quote:
Originally Posted by corey@12mile
Hey Learner... how about this... piss off. you have no idea about originality.
|
haha ... yep, that's original. It was not my intent to be insulting, rather to point out that because of someone's influence sometime in our lives, no one is really original.
Again Tim, thanks for sharing.
|
Offline
|
|
08-10-2005, 07:03 AM
|
#14
|
BlackBerry Extraordinaire
Join Date: Dec 2004
Location: in a house...
Model: lots
Carrier: Rogers
Posts: 1,148
|
Well it's kinda like this... People have to give credit where credit is due. Plain and simple. I understand that people want to protect the code they have written and all, but when you only write 1/3 of the code that you try to copyright, something should be said.
I am not saying that anything is wrong per se, but I am saying give credit to the author/owner. I have posted code a few times, I have also mentioned that entire class's have been copy from other places and given credit.
Tim knows exactly what I am talking about, he writes code for a living, I write code for a living. I have tried several times to spark some interest in posting code up here, but before Tim, nobody else has ever shown interest.
I have 3 projects started which I plan to release under the GPL, and for the last few months I have talked with Guess about the best way to house the projects at BBF, as I really don't like SF.
You tell me that writing code and this and that may be harder than it looks, absolutely it is. But it's paid for my house, my cars and all my toys that I have today. I've wrtten custom blackberry apps for several business's thusfar and think that I have a descent understanding of the whole code thing.
I have offered lots and lots of help to people in here with specific programming questions. Don't try and critisize me as you are not even close to my league.
For a final note, I think it's great that people are starting to post sample code and such, I hope it continues. We just have to make sure that credit is given where it's due, or some author somewhere down the road is gonna complain and make this all for nothing. Copyright is a very serious (legal) thing.
cd.
|
Offline
|
|
08-10-2005, 12:52 PM
|
#15
|
Knows Where the Search Button Is
Join Date: Jun 2005
Model: 7520
Posts: 40
|
Quote:
Originally Posted by corey@12mile
Well it's kinda like this... I have tried several times to spark some interest in posting code up here, but before Tim, nobody else has ever shown interest.
...
cd.
|
Well said.
I do disagree with you on one thing cory. Ahem, *cough* I posted code *cough* before the esteemed timinator.
Very well then. Let's get readdyyyy to coooooOOOOOOODE!
corey@12mile, your project sounds great! Let us know and keep us posted. I am really having a fun and frustrating time working with BB and J2ME. I need all the help I can sniff out.
|
Offline
|
|
08-10-2005, 02:25 PM
|
#16
|
BlackBerry Extraordinaire
Join Date: Dec 2004
Location: in a house...
Model: lots
Carrier: Rogers
Posts: 1,148
|
Quote:
Originally Posted by BlackBerry Fairy
Well said.
I do disagree with you on one thing cory. Ahem, *cough* I posted code *cough* before the esteemed timinator.
Very well then. Let's get readdyyyy to coooooOOOOOOODE!
corey@12mile, your project sounds great! Let us know and keep us posted. I am really having a fun and frustrating time working with BB and J2ME. I need all the help I can sniff out.
|
I don't think I said I posted code before Tim did, I think I said that before Tim started posting code up here, nobody else had ever done anything to that extent. I think I said that I had tried to spark some interest, like end of last year, very early this year in doing something as a group so to speak.
I think there are lots of budding BB programmers out there, and if people don't get their copyrights in a bunch then we could most likely all work together to solve some of these little issues we have.
I think that the notifire software could have been written in less than a month by 3-4 people on here if there was interest. But there was no interest so it's kind of a moot point and now people have to pay for the luxury. I also think a mailbox clone could be written fairly easily with the option to staticly sit on 1 filter setting (ie. inbox only).
I would spearhead projects like this, but management isn't my best thing nor is the whole personal relations thing. I am all for writing some code and helping other people, but I don't have the time, skills or patience to deal with organizing something of this nature.
cd.
|
Offline
|
|
03-05-2007, 06:39 PM
|
#17
|
Knows Where the Search Button Is
Join Date: Nov 2006
Model: 8310
Carrier: AT&T (formerly Cingular)
Posts: 47
|
I know I'm reviving a long dead thread but can anyone tell me what the significance of 0xe63e08aacd575338L is in the code below? Is it a unique number I should create so the system can uniquely assign my data?
Code:
static
{
mystore = PersistentStore.getPersistentObject( 0xe63e08aacd575338L );
}
|
Offline
|
|
03-06-2007, 08:22 AM
|
#18
|
Talking BlackBerry Encyclopedia
Join Date: Jan 2007
Location: Kharkov, Ukraine
Model: 8300
Carrier: N/A
Posts: 237
|
yes. JDE convert string to its hash. Idea is that string is unique enough (includes your company name, software name, etc) that its result hash is unique enough too and nobody will use the same value accidentally.
|
Offline
|
|
03-06-2007, 08:50 AM
|
#19
|
CrackBerry Addict
Join Date: Jun 2005
Location: Manchester, UK
Model: BOLD
Carrier: t-mobile
Posts: 714
|
and relating to what skipper said it's important that the string your hash is created from is unique, so if your persistent object is called namesStore and you create your hash from that - it's not so unique and could potentially cause issues if another developer has a store object by the same name and created his has from it. for this reason it's a good idea to randomize the string your create the hash from, i always use the name of the object followed by some randomly typed numbers, ie.:
//myStore1551535345
//0x770698450c55d247L
myStore = PersistentStore.getPersistentObject(0x770698450c55 d247L);
__________________
new job doesn't allow a public profile - please do not contact this user with questions, you will not get a response. good luck!
|
Offline
|
|
03-08-2007, 03:41 PM
|
#20
|
Talking BlackBerry Encyclopedia
Join Date: Jan 2007
Location: Kharkov, Ukraine
Model: 8300
Carrier: N/A
Posts: 237
|
I didn't see any RIM guidelines for name string which should be used, but from their samples it looks like stick to Sun naming convention for java packages.
I.e. they suppose that if you use "com.<your_company>.<your_organization unit>.<your_product>" string, it should be unique enough
|
Offline
|
|
|
|